Apache Commons DbUtils jar包:commons-dbutils-1.7.jar
下载地址为:https://mvnrepository.com/
1. ResultSetHandler 接口
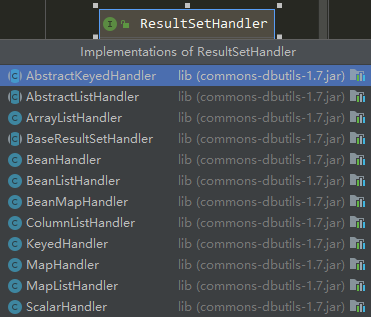
ResultSetHandler 接口的实现类:
- 处理单行数据的类:
ScalarHandler
/ArrayHandler
/MapHandler
/BeanHandler
- 处理多行数据的类:
BeanListHandler
AbstractListHandler抽象类(ArrayListHandler
/MapListHandler
/ColumnListHandler
)
AbstractKeyedHandler抽象类(KeyedHandler
/BeanMapHandler
)
- 可扩展的类:BaseResultSetHandler抽象类(可继承它后实现自己的结果集转换器)
QueryRunner 的 query 方法的返回值最终取决于 query 方法的 ResultHandler 参数的 hanlde 方法的返回值。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package org.apache.commons.dbutils;
import java.sql.ResultSet; import java.sql.SQLException;
public interface ResultSetHandler<T> {
T handle(ResultSet var1) throws SQLException; }
|
2. 单行数据
2.1 ScalarHandler 单值
可以返回指定列的一个值或返回一个统计函数的值,比如count(*)。
该实现类会自动推导数据库中数据的类型,注意类型的转换
1 2 3 4 5 6 7 8 9 10
|
public Integer getTotalSize() throws SQLException { return queryRunner.query( "select count(*) from t_employees", new ScalarHandler<Long>() ).intValue(); }
|
2.2 ArrayHandler 单行对象数组
把结果集中的第一行
数据转成对象数组。
1 2 3 4 5 6 7 8 9 10 11
|
public Object[] getRowData() throws SQLException { return queryRunner.query( "select * from t_employees where id=?", new ArrayHandler(), 10 ); }
|
2.3 MapHandler 单行Map对象
将结果集中的第一行
数据封装到一个Map里,key是列名,value就是对应的值。
1 2 3 4 5 6 7 8 9 10 11
|
public Map<String, Object> getRowData() throws SQLException { return queryRunner.query( "select * from t_employees where id=?", new MapHandler(), 10 ); }
|
2.4 BeanHandler 单行Bean对象
将结果集中的第一行
数据封装到一个对应的JavaBean实例中。
1 2 3 4 5 6 7 8 9 10 11
|
public Employee getSimpleById() throws SQLException { return queryRunner.query( "select * from t_employees where id=?", new BeanHandler<>(Employee.class), 10 ); }
|
3. 多行数据
3.1 ArrayListHandler 多行数组
把结果集中的每一行
数据都转成一个对象数组,再存放到List中。
1 2 3 4 5 6 7 8 9 10
|
public List<Object[]> queryAll() throws SQLException { return queryRunner.query( "select * from t_employees", new ArrayListHandler() ); }
|
3.2 MapListHandler 多行Map
将结果集中的每一行
数据都封装到一个Map里,然后再存放到List。
1 2 3 4 5 6 7 8 9 10
|
public List<Map<String, Object>> queryAll() throws SQLException { return queryRunner.query( "select * from t_employees", new MapListHandler() ); }
|
3.3 ColumnListHandler 单列list
将结果集中某一列
的数据存放到List中,单列多行数据。
1 2 3 4 5 6 7 8 9 10
|
public List<String> queryColumnByName(String colName) throws SQLException { return queryRunner.query( "select " + colName + " from t_employees", new ColumnListHandler<>() ); }
|
3.4 BeanListHandler 多行Bean对象
将结果集中的每一行
数据都封装到一个对应的JavaBean实例中,存放到List里。
1 2 3 4 5 6 7 8 9 10 11 12
|
public List<Employee> queryAllByLimit(int offset, int limit) throws SQLException { return queryRunner.query( "select * from t_employees limit " + offset + "," + limit, new BeanListHandler<>(Employee.class) ); }
|
3.5 KeyedHandler 双层Map
将结果集中的每一行
数据都封装到一个MapA(key是行号,value是行数据)里,再把这些MapA再存到一个MapB(key为指定的列,value是对应里的行值即单值)里
1 2 3 4 5 6 7 8 9 10
|
public Map<String, Map<String, Object>> queryMMapByCol(String colName) throws SQLException { return queryRunner.query( "select * from t_employees", new KeyedHandler<>(colName) ); }
|
3.6 BeanMapHandler
将结果集中的每一行
数据都封装到一个JavaBean里,再把这些JavaBean再存到一个Map里,其key为指定的列。
1 2 3 4 5 6 7 8 9 10
|
public Map<String, Employee> queryMapByCol(String colName) throws SQLException { return queryRunner.query( "select * from t_employees", new BeanMapHandler<>(Employee.class, colName) ); }
|
KeyedHandler 返回的Map的Value是:Map<String, Object>
BeanMapHandler 返回的Map的Value是:JavaBean