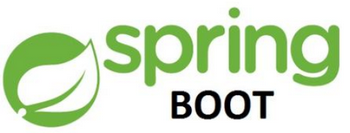
参考资料:https://www.springcloud.cc/spring-boot.html
中文文档2:https://felord.cn/_doc/_springboot/2.1.5.RELEASE/_book/index.html
1. 全局异常处理
SpringBoot 中的异常处理:
1.1 测试类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import com.demo.exception.MyException; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
@RestController @RequestMapping("/ex") public class ExceptionController {
@RequestMapping("/ex1") public void ex1() { int i = 1/0; }
@RequestMapping("/my") public void my() throws MyException { throw new MyException("自定义异常"); } }
|
1.2 自定义异常类
1 2 3 4 5
| public class MyException extends Throwable { public MyException(String msg) { super(msg); } }
|
1.3 全局异常处理类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package com.demo.exception;
import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.RestControllerAdvice;
import javax.servlet.http.HttpServletRequest; import java.util.HashMap;
@RestControllerAdvice public class MyExceptionHandler {
@ExceptionHandler(value = Exception.class) public Object handler1(Exception e, HttpServletRequest request) { System.out.println("handler1");
HashMap<Object, Object> map = new HashMap<>(); map.put("msg", e.getMessage()); map.put("url", request.getRequestURL()); return map; }
@ExceptionHandler(value = MyException.class) public Object handler2(Exception e, HttpServletRequest request) { System.out.println("handler2");
HashMap<Object, Object> map = new HashMap<>(); map.put("msg", e.getMessage()); map.put("url", request.getRequestURL()); return map; } }
|
访问测试:http://localhost:8081/ex/ex1
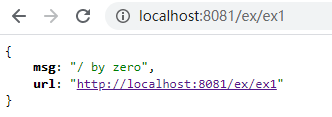
访问测试:http://localhost:8081/ex/my
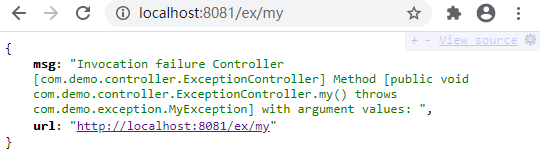
2. 日志
SpringBoot 默认使用的日志是 Logback
,官方建议日志文件命名为:logback-spring.xml
在 resources 目录下创建 logback-spring.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
| <?xml version="1.0" encoding="UTF-8"?>
<configuration scan="true" scanPeriod="60 seconds" debug="true">
<property name="log.path" value="D:/log" /> <property name="LOG_PATH" value="./logs/cache/"/> <property name="CONSOLE_LOG_PATTERN" value="%d{yyyy-MM-dd HH:mm:ss.SSS} |-[%-5p] in %logger.%M[line-%L] -%m%n"/>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<filter class="ch.qos.logback.classic.filter.ThresholdFilter"> <level>debug</level> </filter> <encoder> <Pattern>${CONSOLE_LOG_PATTERN}</Pattern> <charset>UTF-8</charset> </encoder> </appender>
<appender name="file1" class="ch.qos.logback.core.FileAppender"> <file>${log.path}/mylog1.log</file> <encoder> <pattern>${CONSOLE_LOG_PATTERN}</pattern> </encoder> </appender>
<appender name="file2" class="ch.qos.logback.core.rolling.RollingFileAppender"> <file>${log.path}/mylog2.log</file> <encoder> <pattern>${CONSOLE_LOG_PATTERN}</pattern> <charset>UTF-8</charset> </encoder>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy"> <fileNamePattern>${log.path}/newlog-%d{yyyy-MM-dd}.%i.log</fileNamePattern> <timeBasedFileNamingAndTriggeringPolicy class="ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP"> <maxFileSize>1kb</maxFileSize> </timeBasedFileNamingAndTriggeringPolicy> <maxHistory>1</maxHistory> </rollingPolicy> </appender>
<root level="INFO"> <appender-ref ref="CONSOLE"/> <appender-ref ref="file1"/> <appender-ref ref="file2"/> </root> </configuration>
|