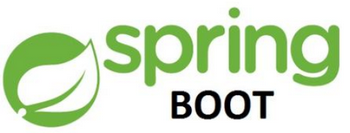
参考资料:https://www.springcloud.cc/spring-boot.html
中文文档2:https://felord.cn/_doc/_springboot/2.1.5.RELEASE/_book/index.html
1. 导入依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.1</version> </dependency>
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
|
2. 配置文件
如果使用 properties 格式的配置:application.properties
1 2 3 4 5 6 7 8 9 10 11 12 13 14
|
spring.datasource.url=jdbc:mysql://localhost:3306/数据库名?serverTimezone=Asia/Shanghai spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.type-aliases-package=com.demo.pojo
mybatis.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
|
如果使用 yml 格式的配置:application.yml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| spring: datasource: username: root password: 123456 url: jdbc:mysql://localhost:3306/数据库名?serverTimezone=Asia/Shanghai driver-class-name: com.mysql.jdbc.Driver type: com.alibaba.druid.pool.DruidDataSource
mybatis: mapper-locations: classpath:com/demo/dao/*.xml type-aliases-package: com.demo.pojo configuration: log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
|
3. 实体类
1 2 3 4 5 6 7 8
| @Data @NoArgsConstructor @AllArgsConstructor public class Account { private Integer id; private String name; private Double money; }
|
4. Mapper
1 2 3 4 5 6 7 8
| import com.demo.pojo.Account; import org.springframework.stereotype.Repository; import java.util.List;
@Repository public interface AccountMapper { public List<Account> findAll(); }
|
5. Mapper.xml
在 src\main\resources\mapper 路径下创建对应的 AccountMapper.xml 文件
1 2 3 4 5 6 7 8 9
| <?xml version="1.0" encoding="utf-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.demo.mapper.AccountMapper"> <select id="findAll" resultType="com.demo.pojo.Account"> select * from account </select> </mapper>
|
6. Service
1 2 3 4 5 6
| import com.demo.pojo.Account; import java.util.List;
public interface AccountService { public List<Account> findAll(); }
|
7. ServiceImpl
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import com.demo.mapper.AccountMapper; import com.demo.pojo.Account; import com.demo.service.AccountService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
import java.util.List;
@Service public class AccountServiceImpl implements AccountService { @Autowired private AccountMapper accountMapper; @Override public List<Account> findAll() { return accountMapper.findAll(); } }
|
8. Controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import com.demo.pojo.Account; import com.demo.service.AccountService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import java.util.List;
@RestController @RequestMapping("/account") public class AccountController { @Autowired private AccountService accountService;
@RequestMapping("/findAll") public List<Account> findAll(){ return accountService.findAll(); } }
|
9. @MapperScan
注意:需要在启动类上添加 @MapperScan
扫描 Mapper
1 2 3 4 5 6 7 8 9 10 11
| import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication @MapperScan("com.demo.mapper") public class Springboot02Application { public static void main(String[] args) { SpringApplication.run(Springboot02Application.class, args); } }
|
10. 分页插件整合
pom.xml
1 2 3 4 5 6
| <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper-spring-boot-starter</artifactId> <version>1.2.13</version> </dependency>
|
Controller 控制器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import com.demo.pojo.Account; import com.demo.service.AccountService; import com.github.pagehelper.PageHelper; import com.github.pagehelper.PageInfo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController @RequestMapping("/account") public class AccountController { @Autowired private AccountService accountService; @RequestMapping("/findByPage") public PageInfo<Account> findAll(@RequestParam(defaultValue = "1") Integer pageNum, @RequestParam(defaultValue = "5") Integer pageSize) {
PageHelper.startPage(pageNum, pageSize); List<Account> accountList = accountService.findAll(); return new PageInfo<>(accountList); } }
|

11. 使用Druid连接池进行测试
11.1 导入依赖
1 2 3 4 5
| <dependency> <groupId>com.alibaba</groupId> <artifactId>druid-spring-boot-starter</artifactId> <version>1.1.10</version> </dependency>
|
11.2 application.properties
1 2
| spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
|
11.3 application.yml
1 2 3 4 5 6 7 8 9 10 11 12 13
| mybatis: type-aliases-package: com.demo.pojo mapper-locations: classpath:mapper/*.xml configuration: log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
spring: datasource: type: com.alibaba.druid.pool.DruidDataSource url: jdbc:mysql://localhost:3306/java2001?serverTimezone=Asia/Shanghai username: root password: root driver-class-name: com.mysql.cj.jdbc.Driver
|