
GitHub地址:https://github.com/Netflix/ribbon
官网地址:https://cloud.spring.io/spring-cloud-static/spring-cloud-netflix/2.1.0.RC2/single/spring-cloud-netflix.html
负载均衡实现:https://www.jb51.net/article/154446.htm
1. Ribbon 简介
Ribbon,是一个客户端的负载均衡
器,它提供对大量的HTTP和TCP客户端的访问控制,可以实现服务的远程调用和服务的负载均衡协调。
7 种负载均衡策略:
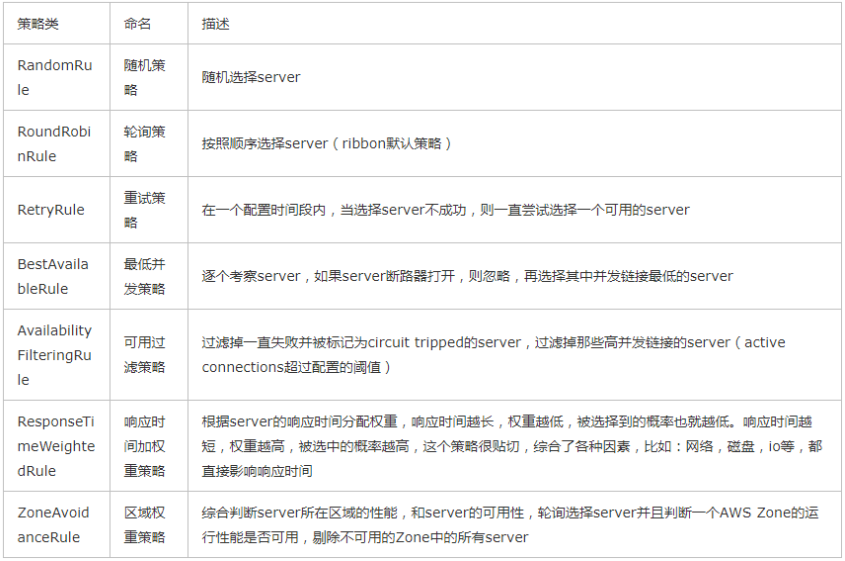
2. Ribbon 开发步骤
- 依赖
1 2 3 4
| <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </dependency>
|
- 编写配置类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| @Configuration public class RibbonConfig { @Bean @LoadBalanced public RestTemplate createRT() { return new RestTemplate(); }
@Bean public IRule createRule() { RandomRule randomRule = new RandomRule(); WeightedResponseTimeRule responseTimeRule = new WeightedResponseTimeRule(); BestAvailableRule bestAvailableRule = new BestAvailableRule(); RoundRobinRule roundRobinRule = new RoundRobinRule(); RetryRule retryRule = new RetryRule(); ZoneAvoidanceRule zoneAvoidanceRule = new ZoneAvoidanceRule(); AvailabilityFilteringRule filteringRule = new AvailabilityFilteringRule(); return randomRule; } }
|
- 启动类配置
1 2 3 4 5 6 7 8
| @SpringBootApplication @EnableDiscoveryClient @RibbonClients public class OfferApiApplication { public static void main(String[] args) { SpringApplication.run(OfferApiApplication.class,args); } }
|
- 接口封装
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| @Api(tags = "用户接口") @RestController @RequestMapping("api/user/") public class UserController { @Autowired private UserService service;
@GetMapping("checknickname/{name}") public R check(@PathVariable String name) { return service.checkN(name); } @PostMapping("register.do") public R save(@RequestBody UserRegisterDto dto){ return service.save(dto); } }
public interface UserService { R checkN(String name); R save(UserRegisterDto dto); }
@Service public class UserServiceImpl implements UserService {
@Autowired private RestTemplate restTemplate;
@Override public R checkN(String name) { return restTemplate.getForObject("http://jerryProvider/provider/user/checkname.do?name=" + name, R.class); }
@Override public R save(UserRegisterDto dto) { return restTemplate.postForObject("http://jerryProvider/provider/user/register.do", dto, R.class); } }
|