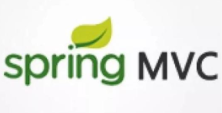
参考资料:https://spring-mvc.linesh.tw/
1. 创建自定义全局异常处理
Controller 调用 service,service 调用 dao,异常都是向上抛出的,最终有 DispatcherServlet 找异常处理器进行异常的处理。
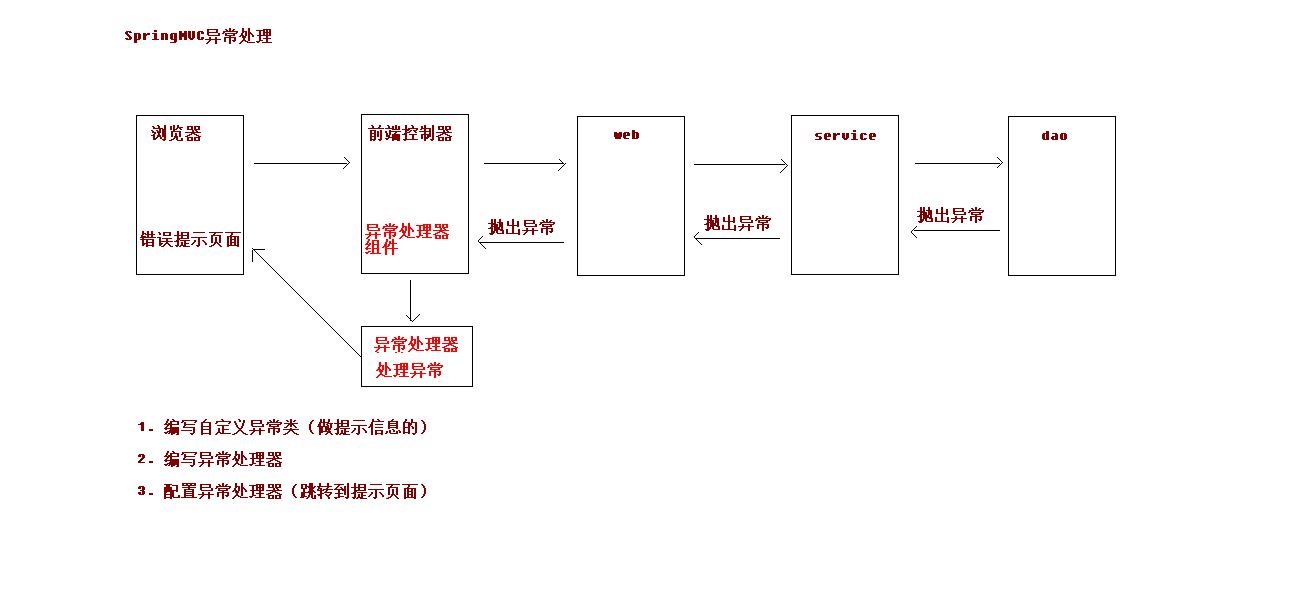
- 创建自定义异常类
1 2 3 4 5
| public class MyException extends Exception { public MyException(String message) { super(message); } }
|
- 创建异常处理器类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class MyExceptionResolver implements HandlerExceptionResolver { @Override public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object object, Exception exception) {
ModelAndView mv = new ModelAndView(); if (exception instanceof MyException) { mv.addObject("msg", "自定义异常:" + exception.getMessage()); } else { mv.addObject("msg", "未知异常"); } mv.setViewName("/error.jsp");
return mv; } }
|
- 在 springmvc.xml 中配置异常处理器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<context:component-scan base-package="com.demo"/>
<bean id="internalResourceViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp"/> </bean>
<mvc:annotation-driven />
<bean id="myExceptionResolver" class="com.demo.ex.MyExceptionResolver"/> </beans>
|
- 在 web.xml 中配置 前端控制器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app> <display-name>Archetype Created Web Application</display-name>
<filter> <filter-name>characterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>characterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
<servlet> <servlet-name>dispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:springmvc.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>dispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
</web-app>
|
- 创建一个 error.jsp 页面,注意
isELIgnored=false
1 2 3 4 5 6 7 8 9 10 11
| <%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>exception</title> </head> <body> <h2>exception</h2> ${msg} </body> </html>
|
- 测试方法
1 2 3 4 5 6 7 8 9 10 11 12
| @Controller @RequestMapping("/ex") public class ExController { @RequestMapping(value="/testException") public void testException() throws MyException{
throw new MyException("自定义异常"); } }
|
本地访问测试:http://localhost:8080/mvc/ex/testEx